I converted the new
Scintillia code edit control IUP example to
C BASIC.
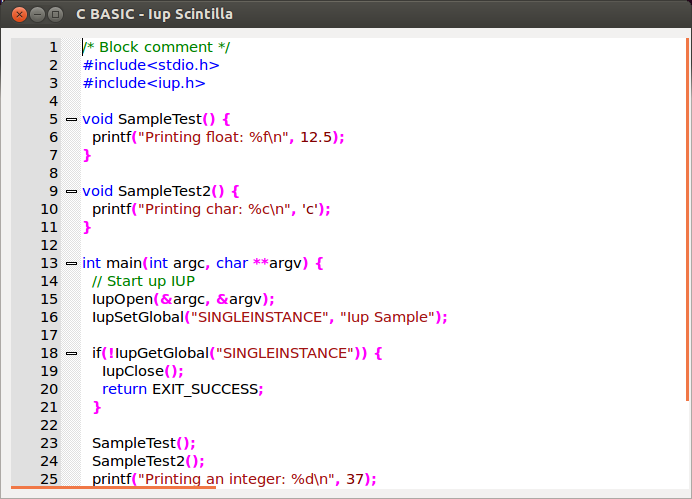
// C BASIC Scintillia edit control example
// gcc iupeditcode.c -I/usr/include/iup -liup -liup_scintilla -o iupeditcode
#include "cbasic.h"
#include <iup.h>
#include <iup_scintilla.h>
DIM AS const char* sampleCode = {
"/* Block comment */\n"
"#include<stdio.h>\n#include<iup.h>\n\n"
"void SampleTest() {\n printf(\"Printing float: %f\\n\", 12.5);\n}\n\n"
"void SampleTest2() {\n printf(\"Printing char: %c\\n\", 'c');\n}\n\n"
"int main(int argc, char **argv) {\n"
" // Start up IUP\n"
" IupOpen(&argc, &argv);\n"
" IupSetGlobal(\"SINGLEINSTANCE\", \"Iup Sample\");\n\n"
" if(!IupGetGlobal(\"SINGLEINSTANCE\")) {\n"
" IupClose(); \n"
" return EXIT_SUCCESS; \n }\n\n"
" SampleTest();\n"
" SampleTest2();\n"
" printf(\"Printing an integer: %d\\n\", 37);\n\n"
" IupMainLoop();\n"
" IupClose();\n"
" return EXIT_SUCCESS;\n}\n"
};
FUNCTION static int marginclick_cb(Ihandle PTR self, int margin, int line, char PTR status)
BEGIN_FUNCTION
PRINT ("MARGINCLICK_CB(Margin: %d, Line: %d, Status:%s)\n", margin, line, status);
PRINT ("Fold Level = %s\n", IupGetAttributeId(self, "FOLDLEVEL", line));
IupSetfAttribute(self, "FOLDTOGGLE", "%d", line);
RETURN IUP_DEFAULT;
END_FUNCTION
FUNCTION static int hotspotclick_cb(Ihandle PTR self, int pos, int line, int col, char PTR status)
BEGIN_FUNCTION
DIM AS char PTR text = IupGetAttributeId(self, "LINE", line);
PRINT ("HOTSPOTCLICK_CB (Pos: %d, Line: %d, Col: %d, Status:%s)\n", pos, line, col, status);
PRINT (" line text = %s\n", text);
RETURN IUP_DEFAULT;
END_FUNCTION
FUNCTION static int button_cb(Ihandle PTR self, int button, int pressed, int x, int y, char PTR status)
BEGIN_FUNCTION
PRINT ("BUTTON_CB = button: %d, pressed: %d, x: %d, y: %d, status: %s\n", button, pressed, x, y, status);
(void)self;
RETURN IUP_DEFAULT;
END_FUNCTION
FUNCTION static int motion_cb(Ihandle PTR self, int x, int y, char PTR status)
BEGIN_FUNCTION
PRINT ("MOTION_CB = x: %d, y: %d, status: %s\n", x, y, status);
(void)self;
RETURN IUP_DEFAULT;
END_FUNCTION
FUNCTION static int caret_cb(Ihandle PTR self, int lin, int col, int pos)
BEGIN_FUNCTION
PRINT ("CARET_CB = lin: %d, col: %d, pos: %d\n", lin, col, pos);
(void)self;
RETURN IUP_DEFAULT;
END_FUNCTION
FUNCTION static int valuechanged_cb(Ihandle PTR self)
BEGIN_FUNCTION
PRINT ("VALUECHANGED_CB\n");
(void)self;
RETURN IUP_DEFAULT;
END_FUNCTION
FUNCTION static int action_cb(Ihandle PTR self, int insert, int pos, int length, char PTR text)
BEGIN_FUNCTION
PRINT ("ACTION = insert: %d, pos: %d, lenght:%d, text: %s\n", insert, pos, length, text);
(void)self;
RETURN IUP_IGNORE;
END_FUNCTION
FUNCTION static void set_attribs (Ihandle PTR sci)
BEGIN_FUNCTION
IupSetAttribute(sci, "CLEARALL", "");
IupSetAttribute(sci, "LEXERLANGUAGE", "cpp");
IupSetAttribute(sci, "KEYWORDS0", "void struct union enum char short int long double float signed unsigned const static extern auto register volatile bool class private protected public friend inline template virtual asm explicit typename mutable"
"if else switch case default break goto return for while do continue typedef sizeof NULL new delete throw try catch namespace operator this const_cast static_cast dynamic_cast reinterpret_cast true false using"
"typeid and and_eq bitand bitor compl not not_eq or or_eq xor xor_eq");
IupSetAttribute(sci, "STYLEFONT32", "Consolas");
IupSetAttribute(sci, "STYLEFONTSIZE32", "11");
IupSetAttribute(sci, "STYLECLEARALL", "Yes"); /* sets all styles to have the same attributes as 32 */
IupSetAttribute(sci, "STYLEFGCOLOR1", "0 128 0"); // 1-C comment
IupSetAttribute(sci, "STYLEFGCOLOR2", "0 128 0"); // 2-C++ comment line
IupSetAttribute(sci, "STYLEFGCOLOR4", "128 0 0"); // 4-Number
IupSetAttribute(sci, "STYLEFGCOLOR5", "0 0 255"); // 5-Keyword
IupSetAttribute(sci, "STYLEFGCOLOR6", "160 20 20"); // 6-String
IupSetAttribute(sci, "STYLEFGCOLOR7", "128 0 0"); // 7-Character
IupSetAttribute(sci, "STYLEFGCOLOR9", "0 0 255"); // 9-Preprocessor block
IupSetAttribute(sci, "STYLEFGCOLOR10", "255 0 255"); // 10-Operator
IupSetAttribute(sci, "STYLEBOLD10", "YES");
// 11-Identifier
IupSetAttribute(sci, "STYLEHOTSPOT6", "YES");
IupSetAttribute(sci, "INSERT0", sampleCode);
IupSetAttribute(sci, "MARGINWIDTH0", "50");
IF (1) THEN
IupSetAttribute(sci, "PROPERTY", "fold=1");
IupSetAttribute(sci, "PROPERTY", "fold.compact=0");
IupSetAttribute(sci, "PROPERTY", "fold.comment=1");
IupSetAttribute(sci, "PROPERTY", "fold.preprocessor=1");
IupSetAttribute(sci, "MARGINWIDTH1", "20");
IupSetAttribute(sci, "MARGINTYPE1", "SYMBOL");
IupSetAttribute(sci, "MARGINMASKFOLDERS1", "Yes");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDER=PLUS");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDEROPEN=MINUS");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDEREND=EMPTY");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDERMIDTAIL=EMPTY");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDEROPENMID=EMPTY");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDERSUB=EMPTY");
IupSetAttribute(sci, "MARKERDEFINE", "FOLDERTAIL=EMPTY");
IupSetAttribute(sci, "FOLDFLAGS", "LINEAFTER_CONTRACTED");
IupSetAttribute(sci, "MARGINSENSITIVE1", "YES");
END_IF
PRINT ("Number of chars in this text: %s\n", IupGetAttribute(sci, "COUNT"));
PRINT ("Number of lines in this text: %s\n", IupGetAttribute(sci, "LINECOUNT"));
PRINT ("%s\n", IupGetAttribute(sci, "LINEVALUE"));
END_FUNCTION
SUB ScintillaTest()
BEGIN_SUB
DIM AS Ihandle PTR dlg, PTR sci;
IupScintillaOpen();
// Creates an instance of the Scintilla control
sci = IupScintilla();
IupSetAttribute(sci, "EXPAND", "Yes");
IupSetCallback(sci, "MARGINCLICK_CB", (Icallback)marginclick_cb);
IupSetCallback(sci, "HOTSPOTCLICK_CB", (Icallback)hotspotclick_cb);
IupSetCallback(sci, "CARET_CB", (Icallback)caret_cb);
IupSetCallback(sci, "VALUECHANGED_CB", (Icallback)valuechanged_cb);
IupSetCallback(sci, "ACTION", (Icallback)action_cb);
// Creates a dialog containing the control
dlg = IupDialog(IupVbox(sci, NULL));
IupSetAttribute(dlg, "TITLE", "C BASIC - Iup Scintilla");
IupSetAttribute(dlg, "RASTERSIZE", "700x500");
IupSetAttribute(dlg, "MARGIN", "10x10");
// Shows dialog
IupShow(dlg);
IupSetAttribute(dlg, "RASTERSIZE", NULL);
set_attribs(sci);
END_SUB
MAIN
BEGIN_FUNCTION
IupOpen(AT argc, AT argv);
ScintillaTest();
IupMainLoop();
IupClose();
RETURN EXIT_SUCCESS;
END_FUNCTION
jrs@laptop:~/C_BASIC/iup$ ./iupeditcode
Number of chars in this text: 527
Number of lines in this text: 31
/* Block comment */
CARET_CB = lin: 1, col: 0, pos: 20
CARET_CB = lin: 2, col: 0, pos: 38
CARET_CB = lin: 3, col: 0, pos: 54
ACTION = insert: 1, pos: 54, lenght:1, text: #
VALUECHANGED_CB
CARET_CB = lin: 3, col: 1, pos: 55
ACTION = insert: 0, pos: 54, lenght:1, text: (null)
VALUECHANGED_CB
CARET_CB = lin: 3, col: 0, pos: 54