This is a IUP button example showing how button events are captured, adding an image (from code) that toggles with the button state. The second image shows the button disabled. The
big useless button state is printed to the console. (left/center[wheel]/right) Notice that the title bar system buttons are also disable (hidden) along with the ability to resize the window.
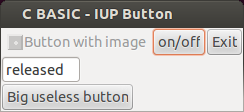
// C BASIC - IUP Button
// gcc button.c -I/usr/include/iup -liup -o button
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <iup.h>
#include "cbasic.h"
DIM AS static unsigned char pixmap_release[] =
{
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,4,4,3,3,3,3,2,2,
1,1,3,3,3,3,3,4,4,4,4,3,3,3,2,2,
1,1,3,3,3,3,3,4,4,4,4,3,3,3,2,2,
1,1,3,3,3,3,3,3,4,4,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,
2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2
};
DIM AS static unsigned char pixmap_press[] =
{
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,4,4,3,3,3,3,3,2,2,
1,1,3,3,3,3,4,4,4,4,3,3,3,3,2,2,
1,1,3,3,3,3,4,4,4,4,3,3,3,3,2,2,
1,1,3,3,3,3,3,4,4,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,
2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2
};
DIM AS static unsigned char pixmap_inactive[] =
{
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,
1,1,1,1,1,1,1,1,1,1,1,1,1,1,1,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,4,4,3,3,3,3,2,2,
1,1,3,3,3,3,3,4,4,4,4,3,3,3,2,2,
1,1,3,3,3,3,3,4,4,4,4,3,3,3,2,2,
1,1,3,3,3,3,3,3,4,4,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,1,3,3,3,3,3,3,3,3,3,3,3,3,2,2,
1,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,
2,2,2,2,2,2,2,2,2,2,2,2,2,2,2,2
};
CB_FUNCTION btn_on_off_cb(Ihandle PTR self)
BEGIN_FUNCTION
DIM AS Ihandle PTR btn_image;
btn_image = IupGetHandle("btn_image");
IF (NOT strcmp(IupGetAttribute(btn_image, "ACTIVE"), "YES")) THEN
IupSetAttribute(btn_image, "ACTIVE","NO");
ELSE
IupSetAttribute(btn_image, "ACTIVE", "YES");
END_IF
RETURN_FUNCTION(IUP_DEFAULT);
END_FUNCTION
CB_FUNCTION btn_image_button_cb(Ihandle PTR self, int b, int e)
BEGIN_FUNCTION
IF (b EQ IUP_BUTTON1) THEN
DIM AS Ihandle PTR text;
text = IupGetHandle("text");
IF (e EQ 1) THEN
IupSetAttribute(text, "VALUE", "pressed");
ELSE
IupSetAttribute(text, "VALUE", "released");
END_IF
END_IF
RETURN_FUNCTION(IUP_DEFAULT);
END_FUNCTION
CB_FUNCTION btn_big_button_cb(Ihandle PTR self, int button, int press)
BEGIN_FUNCTION
printf("BUTTON_CB(button=%c, press=%d)\n", button, press);
RETURN_FUNCTION(IUP_DEFAULT);
END_FUNCTION
CB_FUNCTION btn_exit_cb(Ihandle PTR self)
BEGIN_FUNCTION
RETURN_FUNCTION(IUP_CLOSE);
END_FUNCTION
MAIN
BEGIN_FUNCTION
DIM AS Ihandle PTR dlg,
PTR btn_image,
PTR btn_exit,
PTR btn_big,
PTR btn_on_off,
PTR img_release,
PTR img_press,
PTR img_inactive,
PTR text;
IupOpen(AT argc, AT argv);
IupSetGlobal("UTF8MODE", "Yes");
text = IupText(NULL);
IupSetAttribute(text, "READONLY", "YES");
IupSetHandle ("text", text);
img_release = IupImage(16, 16, pixmap_release);
IupSetAttribute(img_release, "1", "215 215 215");
IupSetAttribute(img_release, "2", "40 40 40");
IupSetAttribute(img_release, "3", "30 50 210");
IupSetAttribute(img_release, "4", "240 0 0");
IupSetHandle("img_release", img_release);
img_press = IupImage(16, 16, pixmap_press);
IupSetAttribute(img_press, "1", "40 40 40");
IupSetAttribute(img_press, "2", "215 215 215");
IupSetAttribute(img_press, "3", "0 20 180");
IupSetAttribute(img_press, "4", "210 0 0");
IupSetHandle ("img_press", img_press);
img_inactive = IupImage(16, 16, pixmap_inactive);
IupSetAttribute(img_inactive, "1", "215 215 215");
IupSetAttribute(img_inactive, "2", "40 40 40");
IupSetAttribute(img_inactive, "3", "100 100 100");
IupSetAttribute(img_inactive, "4", "200 200 200");
IupSetHandle ("img_inactive", img_inactive);
btn_image = IupButton ("Button with image", "btn_image");
IupSetAttribute(btn_image, "IMAGE", "img_release");
IupSetAttribute(btn_image, "IMPRESS", "img_press");
IupSetAttribute(btn_image, "IMINACTIVE", "img_inactive");
IupSetAttribute(btn_image, "BUTTON_CB", "btn_image_button");
IupSetHandle("btn_image", btn_image);
btn_big = IupButton("Big useless button", "");
IupSetAttribute(btn_big, "SIZE", "EIGHTHxEIGHTH");
btn_exit = IupButton("Exit", "btn_exit");
btn_on_off = IupButton("on/off", "btn_on_off");
dlg = IupDialog
(
IupVbox
(
IupHbox
(
btn_image,
btn_on_off,
btn_exit,
NULL
),
text,
btn_big,
NULL
)
);
IupSetAttributes(dlg, "EXPAND = YES, TITLE = \"C BASIC - IUP Button\", RESIZE = NO");
IupSetAttributes(dlg, "MENUBOX = NO, MAXBOX = NO, MINBOX = NO");
IupSetCallback(btn_exit, "ACTION", (Icallback) btn_exit_cb);
IupSetCallback(btn_on_off, "ACTION", (Icallback) btn_on_off_cb);
IupSetCallback(btn_image, "BUTTON_CB", (Icallback) btn_image_button_cb);
IupSetAttribute(btn_big, "BUTTON_CB", "bigtest");
IupSetFunction("bigtest", (Icallback)btn_big_button_cb);
IupShowXY(dlg, IUP_CENTER, IUP_CENTER);
IupMainLoop();
IupClose();
RETURN_FUNCTION(EXIT_SUCCESS);
END_FUNCTION
jrs@laptop:~/C_BASIC/iup$ ./button
BUTTON_CB(button=1, press=1)
BUTTON_CB(button=1, press=0)
BUTTON_CB(button=2, press=1)
BUTTON_CB(button=2, press=0)
BUTTON_CB(button=3, press=1)
BUTTON_CB(button=3, press=0)
jrs@laptop:~/C_BASIC/iup$
The next example in this series is how IUP places its controls using its containers and controlling auto sizing of controls. I'm going to have to create a custom demo as these features are currently spread out over a few examples.