I thought I would try doing the
graphdemo using
C BASIC. I have created a work in progress version of the BBC BASIC graphics library which I'm calling
libbbc5.c. (original libbbc.c is still on Bitbucket) The goal is a generic BBC BASIC graphics plug-in any language can bind to without having any SDL knowledge per say. It will handle the keyboard / mouse events along with a few BBC BASIC specific functions (BBC_RND, BBC_TIME, ...) to help port BBC programs to the language of your choice. I plan to convert the existing examples to
C BASIC and post them on the Bitbucket site.
Update: I added BBC_WAITKEY, BBC_GETKEY and BBC_RND to the
libbbc5 library. The most current version is on Bitbucket if you're following along.
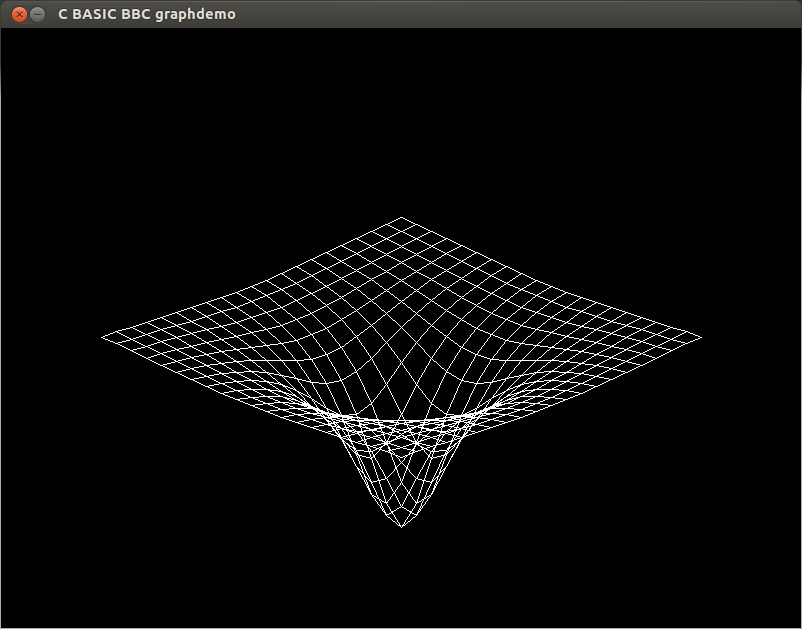
// Graph Demo
#include <SDL.h>
#include "libbbc5.h"
#include "cbasic.h"
MAIN
BEGIN_FUNCTION
DIM AS long xlow, xhigh, ylow, yhigh, depth, xscale, yscale, c, x, y;
BBC_OPEN();
SDL_WM_SetCaption("C BASIC BBC graphdemo", 0);
BBC_MODE(31);
BBC_ORIGIN(800, 600);
xlow = -10;
xhigh = 10;
ylow = -10;
yhigh = 10;
depth = 10;
xscale = 30;
yscale = 12;
c = -4000;
x = 0;
y = 0;
DEF_FOR (x = xlow TO x <= xhigh STEP x += 1)
BEGIN_FOR
BBC_MOVE(xscale*(x+ylow), yscale*(ylow-x)+c/(x*x+ylow*ylow+depth));
DEF_FOR (y = ylow TO y <= yhigh STEP y += 1)
BEGIN_FOR
BBC_DRAW(xscale*(x+y), yscale*(y-x)+c/(x*x+y*y+depth));
NEXT
NEXT
DEF_FOR (y = ylow TO y <= yhigh STEP y += 1)
BEGIN_FOR
BBC_MOVE(xscale*(xlow+y), yscale*(y-xlow)+c/(xlow*xlow+y*y+depth));
DEF_FOR (x = xlow TO x <= xhigh STEP x += 1)
BEGIN_FOR
BBC_DRAW(xscale*(x+y), yscale*(y-x)+c/(x*x+y*y+depth));
NEXT
NEXT
// BBC_WAITKEY();
DEF_WHILE (BBC_GETKEY() != SDLK_ESCAPE)
BEGIN_WHILE
WEND
BBC_CLOSE();
RETURN_FUNCTION(0);
END_FUNCTION
This is the
libbbc.dll version running under Windows with ScriptBasic and DLLC.
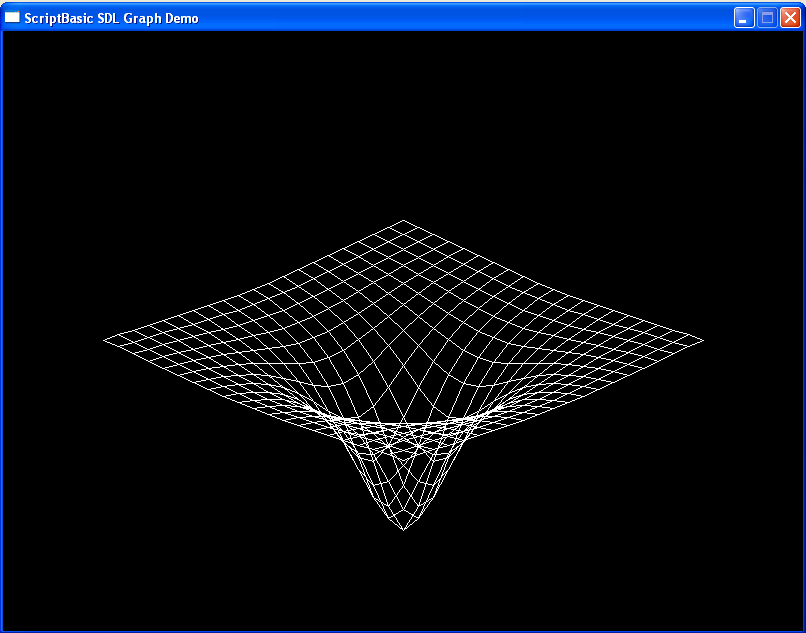
' Graph Demo
INCLUDE "dllcinc.sb"
bbc = dllfile("libbbc.dll")
sdl = dllfile("sdl.dll")
BBC_OPEN = dllproc(bbc,"init_screen i = ()")
BBC_MODE = dllproc(bbc,"emulate_mode (i mode)")
BBC_ORIGIN = dllproc(bbc,"emulate_origin (i x, i y)")
BBC_DRAW = dllproc(bbc,"emulate_draw (i x, i y)")
BBC_MOVE = dllproc(bbc,"emulate_move (i x, i y)")
BBC_CLOSE = dllproc(bbc,"end_screen ()")
SDL_WINTITLE = dllproc(sdl,"SDL_WM_SetCaption (z title, z icon)")
SDL_WAIT = dllproc(sdl,"SDL_Delay (i millsecs)")
dllcall BBC_OPEN
dllcall SDL_WINTITLE, "ScriptBasic SDL Graph Demo", "ScriptBasic"
dllcall BBC_MODE, 31
dllcall BBC_ORIGIN, 800, 600
xlow = -10
xhigh = 10
ylow = -10
yhigh = 10
depth = 10
xscale = 30
yscale = 12
c = -4000
FOR x = xlow TO xhigh
dllcall BBC_MOVE, xscale*(x+ylow), yscale*(ylow-x)+c/(x*x+ylow*ylow+depth)
FOR y = ylow TO yhigh
dllcall BBC_DRAW, xscale*(x+y), yscale*(y-x)+c/(x*x+y*y+depth)
NEXT
NEXT
FOR y = ylow TO yhigh
dllcall BBC_MOVE, xscale*(xlow+y), yscale*(y-xlow)+c/(xlow*xlow+y*y+depth)
FOR x = xlow TO xhigh
dllcall BBC_DRAW, xscale*(x+y), yscale*(y-x)+c/(x*x+y*y+depth)
NEXT
NEXT
dllcall SDL_WAIT, 5000
dllcall BBC_CLOSE